Javascript Tools in Action
Discover some retrieving JavaScript tools to level up your programming game. Learn more about ESLint, Prettier and Husky!
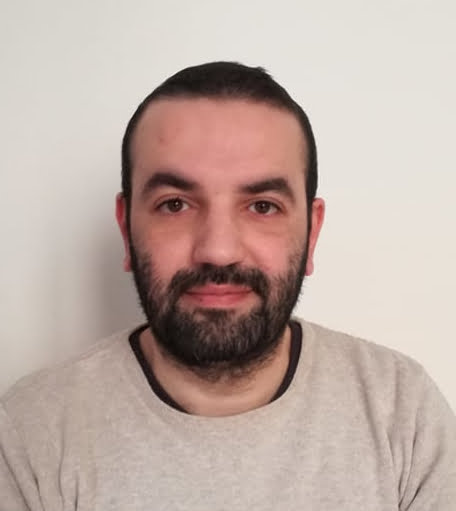
Why you shouldn’t be using Options API in Due? Find answer to this question in the following article and discover the benefits of Composition API.
Vue 3 introduced a new approach to creating components called Composition API
. It is an alternative to the Options API
. The Composition API is fully optional and you don’t need to use it if you want to use Vue 3. However, it introduces some important improvements that make your work easier and improve the readability of the code.
The Composition API introduces a new method called setup
. Inside it, you can create all the necessary elements of the component, such as: data
, methods
, computed properties
, watchers
. Thanks to this, you can keep the code clean by placing the logic responsible for a given feature in one place. In contrast, the Options API forced the scattering of the logic throughout the file. As a result, the code was not readable and required scrolling. In Composition API methods, watchers etc. no longer need to be grouped by type, you can put them as you see fit.
The Composition API allows you to write reactive code anywhere. You can pull some reactive logic outside of the component. This type of code is called Composables
. Composables can be imported into many components and allow you to encapsulate some logic and expose the necessary reactive elements.
// shopping-list.js
import ( computed ) from "vue";
export function useShoppingList(listId) (
const products = shoppingList.getAllProducts(listId);
const productsCount = computed(() => products.value.length);
const deleteProduct = (productId) => shoppingList.deleteProduct(productId);
return (
products,
productsCount,
deleteProduct,
);
)
// Component
import useSpoppingList from "@/composables/shopping-list.js";
export default (
setup() (
const ( products, productsCount, deleteProduct ) = useSpoppingList();
return ( products, productsCount, deleteProduct );
),
);
In the Options API, to add e.g. a static list of items imported from another file, you need to add it to data() (which has a negative impact on performance) or add it to this
in created()
. Both ways are not very elegant. The Composition API and the new setup
method come to the rescue, from which you can export not only reactive things but also constants and external dependencies.
import list from "./list.json";
export default (
setup() (
return ( list );
),
);
Thanks to the official @vue/composition-api
plugin, you can use Composition API in Vue 2 as well.
<h3>Setup method</h3>
setup() is a new method added in Vue 3 where you can put all the necessary items such as data objects, methods etc. From there you can return the elements you want to include in the template.
<template>
<div>(( count ))</div>
</template>
import ( ref ) from "vue";
export default (
setup() (
const count = ref(10);
return ( count );
),
);
To create a reactive object or array you have to create it with the reactive(defaultValue)
method. You can pass the initial value of this object via a method argument. The method returns a proxy
for this object, so when you make changes to it, Vue knows about them and can properly refresh the view.
Composition API
import ( reactive ) from "vue";
export default (
setup() (
const user = reactive((
name: "John",
role: "ADMIN",
));
return ( user );
),
);
Options API
export default (
data() (
return (
user: (
name: "John",
role: "ADMIN",
),
);
),
);
Reactive only works for object types (objects, arrays, and collection types such as Map
and Set
). It cannot hold primitive types such as string
, number
or boolean
. So Vue also introduces ref()
.
To add reactivity to primitive elements you have to wrap them with ref()
.
Composition API
import ( ref ) from "vue";
export default (
setup() (
const count = ref(10);
return ( count );
),
);
Options API
export default (
data() (
return (
count: 10,
);
),
);
You can change values in objects from the reactive method directly, but to change primitive values you must use the .value
field.
import ( ref, reactive ) from "vue";
export default (
setup() (
const user = reactive((
name: "John",
));
user.name = "John Doe"; // Value change
const count = ref(10);
count.value = 20; // Value change
return (
user,
count,
);
),
);
You don’t need to use the .value
property in the template.
<div>(( count ))</div>
Computed properties can be easily created by passing a method as a parameter to the imported computed()
method.
import ( reactive, computed ) from "vue";
export default (
setup() (
const list = reactive([
"Item 1",
"Item 2",
]);
// Computed
const isEmpty = computed(() => list.length === 0);
return (
list,
isEmpty,
);
),
);
You can also nest methods in the setup
method.
Composition API
import ( ref ) from "vue";
export default (
setup() (
const count = ref(10);
// Method
const increment = () => (
count.value++;
);
return (
count,
increment,
);
),
);
Watchers are created in a similar way to computed
. However, remember to pass the proxy
object
, i.e. name
, as in the example below, not the name.value
value itself.
import ( watch, ref ) from "vue";
export default (
setup() (
const name = ref("John");
// Watcher
watch(name, (currentValue, oldValue) => (
console.log(`Value changed from $(oldValue)to $(currentValue)`);
));
return ( name );
),
);
In this article, I have only given you the basics of the Composition API so that you have a general understanding of how it differs and what advantages it has compared to the Options API. The Composition API also provides alternatives to the rest of the component’s elements, such as hooks
, and introduces new methods, such as watchEffect
. It is a good idea to read it in the official Vue 3 documentation.