9 Mistakes to Avoid While Programming in Java
What mistakes should be avoided while programming in Java? In the following piece we answers this question.
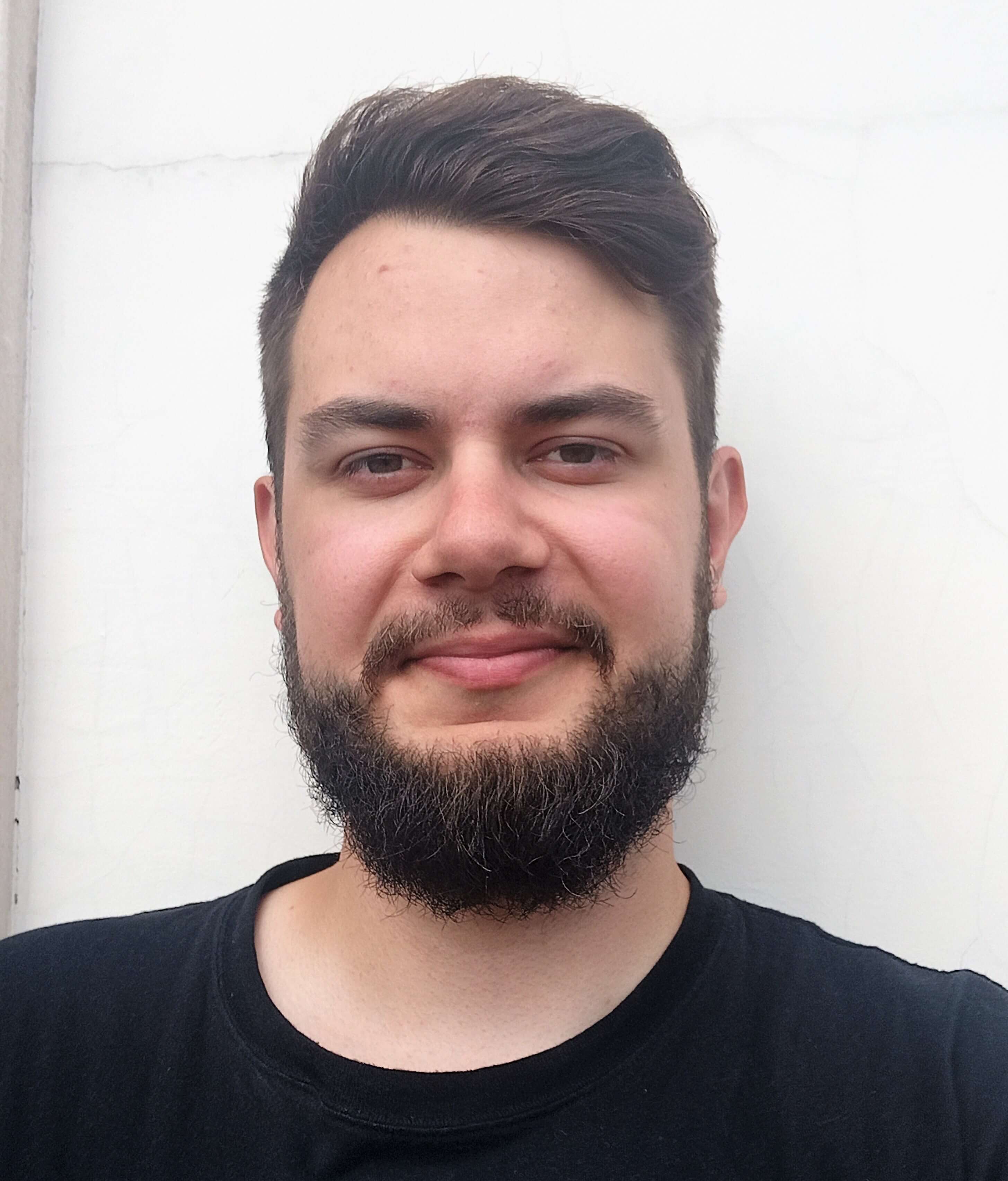
Learn how to run containers form tests in our Java related article where our senior java developer shows all the magic.
I use Spring Boot in the project to reduce boilerplate. Test containers are independent of Spring Framework and you can use them without that.
I use Testcontainers version 1.17.3, but feel free to use the newest one. Tests with Postgres container.
First define container:
public class Postgres13TC extends PostgreSQLContainer<Postgres13TC> {
private static final Postgres13TC TC = new Postgres13TC();
private Postgres13TC() {
super("postgres:13.2");
}
public static Postgres13TC getInstance() {
return TC;
}
@Override
public void start() {
super.start();
System.setProperty("DB_URL", TC.getJdbcUrl());
System.setProperty("DB_USERNAME", TC.getUsername());
System.setProperty("DB_PASSWORD", TC.getPassword());
}
@Override
public void stop() {
// do nothing. This is shared instance. Let JVM handle this operation.
}
}
Then initialize Spring application context. We get all data source configuration from container instance and set them as Spring configuration:
public class ContainerInit implements ApplicationContextInitializer<ConfigurableApplicationContext> {
public static Postgres13TC postgres13TC;
static {
postgres13TC = Postgres13TC.getInstance();
postgres13TC.start();
}
@Override
public void initialize(ConfigurableApplicationContext applicationContext) {
TestPropertySourceUtils.addInlinedPropertiesToEnvironment(
applicationContext,
"spring.datasource.url=" + postgres13TC.getJdbcUrl(),
"spring.datasource.username=" + postgres13TC.getUsername(),
"spring.datasource.password=" + postgres13TC.getPassword(),
"db.host=" + postgres13TC.getHost(),
"db.port=" + postgres13TC.getMappedPort(postgres13TC.POSTGRESQL_PORT),
"db.name=" + postgres13TC.getDatabaseName(),
"db.username=" + postgres13TC.getUsername(),
"db.password=" + postgres13TC.getPassword()
);
}
}
Finally, we can run our test, and everything will be started under the hood:
@SpringBootTest(webEnvironment = RANDOM_PORT)
@AutoConfigureTestDatabase(replace = NONE)
@ContextConfiguration(initializers = ContainerInit.class)
@Testcontainers
class DummyRepositoryTest {
@Autowired
private DummyRepository dummyRepository;
@Test
void shouldReturnDummy() {
var byId = dummyRepository.getById(10L);
var expected = new Dummy();
expected.setId(10L);
assertThat(byId).completes().emitsCount(1).emits(expected);
}
}
Or if we want to run Spring independent test, we can use container directly:
@Testcontainers
class SimpleDbTest {
@Container
private static final Postgres13TC postgres13TC = Postgres13TC.getInstance();
@Test
void testConnection() {
assumeThat(postgres13TC.isRunning());
var connectionProps = new Properties();
connectionProps.put("user", postgres13TC.getUsername());
connectionProps.put("password", postgres13TC.getPassword());
try (Connection connection = DriverManager.getConnection(postgres13TC.getJdbcUrl(),
connectionProps)) {
var resultSet = connection.prepareStatement("Select 1").executeQuery();
resultSet.next();
assertThat(resultSet.getInt(1)).isEqualTo(1);
} catch (SQLException sqlException) {
assertThat((Exception) sqlException).doesNotThrowAnyException();
}
}
}
Testcontainers are very easy-to-use tools that help us to create integration tests that use Docker containers. That gives us more flexibility and increases development speed. Proper setup of test configuration reduces the time needed to board new developers. They don’t need to set up all dependencies, just run the written tests with selected configuration files.