Vue.js app improvement. Some practical tips
Vue is a rapidly growing progressive framework for building user interfaces. It became the JavaScript framework with the most stars on GitHub and the most highly starred project of 2016...
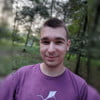
Nuxt 3 is the next generation of the popular hybrid Vue framework, which allows us to use Vue for building server-side rendered applications. Beta version was launched on 12 October 2021, bringing into Nuxt Vue 3, a new intro engine, a lighter bundle and adhook Vite.
Nuxt 3 has been re-architected and re-written to support ESM and TypeScript natively. It’s currently unstable, which makes it not yet production-ready. The first candidate ([email protected]) is planned to be released on 7 April 2022.
Open a terminal or open an integrated terminal from Visual Studio Code and use the following command to create a new starter project:
npx nuxi init nuxt3-app
Open the nuxt3-app folder:
cd nuxt3-app
Install the dependencies:
yarn install
Run the development server:
yarn dev
Build the application:
yarn build
Run the built application:
yarn start
Vue 3 came with several new features that make building and maintaining applications a lot faster and easier. The most important changes are made to Global Vue API and Events API. Vue 3also introduces new features like provide / inject, composition API (described below), Fragments, and Teleport.
Composition API is a built-in Vue 3 feature, which provides a set of APIs, which allows the use of imported functions instead of declaring options. So, the main advantages of Composition API are better logic reuse, more flexible code organization and great TypeScript integration. All parts of the new API can be used outside of Vue components.
Nuxt 3 provides a new directory – composables/
– that allows to auto-import Vue composables intro application.
Example composable:
// It will be available as useFoo() (camelCase of file name without extension)
export default function () {
return useState('foo', () => 'bar')// It will be available as useFoo() (camelCase of file name without extension)
export default function () {
return useState('foo', () => 'bar')
}
}
Example of using a composable in a Vue component:
<div>{{ foo }}</div>
</>
As you can see above, a composable is exported as useFoo, just as declared in the const name. If there is no export name, the composable will be accessible as pascelCase of the file name. It also allows you to easily integrate auto-imported composables with a popular Vue Store composable called Pina.
Nitro is a full-stack server which uses Rollup and Node.js workers in development to serve code and context isolation. It also includes a server API and server middleware. In production, the engine builds the application and the server into one directory – `.output`. The thing is that output is lightweight: minified and without any node modules. Nitro allows you to deploy the output on Node.js, Serverless, Workers, Edge-side rendering, or as purely static.
Nuxt 3 provides the possibility to deploy on Azure or Netlify with no configuration required, along with a Firebase or Cloudflare deploy with minimal configuration.
Vite is a next-generation frontend tooling, which is a built-in feature for Nuxt 3. This tool provides a faster development experience for web projects. For development, the server Vite has rich feature enhancements over native ES modules and a very fast Hot Module Replacement (HMR).
In the building process, Vite bundles code with preconfigured Rollup to optimize static assets for production.
Nuxt 3 introduces a slightly changed file structure. The biggest changes were done to `app.vue` – `pages/` directory is optional and if isn’t present, the application won’t include a vue-router, which is useful when creating a loading page or an application that doesn’t need routing.
Example app.vue file:
Hello World!
To include routing, pages and layout, you need to use built-in components, namely `NuxtPage` and `NuxtLayout`. Example:
<div>
<p> </p>
<p> </p>
<p> </p>
</div>
`app.vue` is the main component of the Nuxt application, so everything added there will be global and included in every page.
The change in the Nuxt directory structure involves replacing `store/` with `composables/` directory, since Vue 3introduces composables which replaces stores.
Nuxt 3 introduces Nuxt Bridge – forward-compatibility layer that upgrades Nuxt 2 applications with Nuxt 3 features, allowing it to upgrade itself piece by piece. It provides access to features like: Nitro engine, composition API and new CLI, by simply installing and activating the bridge.
Nuxt Bridge is backward compatible, so legacy plugins and modules will still work, while migrating is easy and possible without rewriting the whole application.
To enable Nuxt Bridge, you have to make sure that the dev server isn’t running, then remove any package lock files and install `nuxt-edge`:
- "nuxt": "^2.15.0"
"nuxt-edge": "latest"
After that, reinstall all dependencies:
yarn install
Your migration using Nuxt Bridge is done!
Another way is to install Nuxt Bridge as a development dependency:
yarn add --dev @nuxt/bridge@npm:@nuxt/bridge-edge
Then you have to update scripts in `package.json` to take into account changes that the Nitro server brings to the building process.