“Don`t block the event loop…” – you’ve probably heard this sentence many times… I’m not surprised because it is one of the most important assumptions when working with Node. But there is also a second “thing” which you should refrain from blocking – the Worker Pool. If neglected, it can have a significant impact on application performance and even its security.
Threads
Main thing to remember: there are two types of threads in Node.js: Main Thread – which is handled by Event Loop, and Worker Pool (thread pool) – which is the pool of threads –
thanks to libuv. Each of them has a different job. The goal of the first one is to handle non-blocking I/O operations, and the second is responsible for CPU intensive work and also blocking I/O.
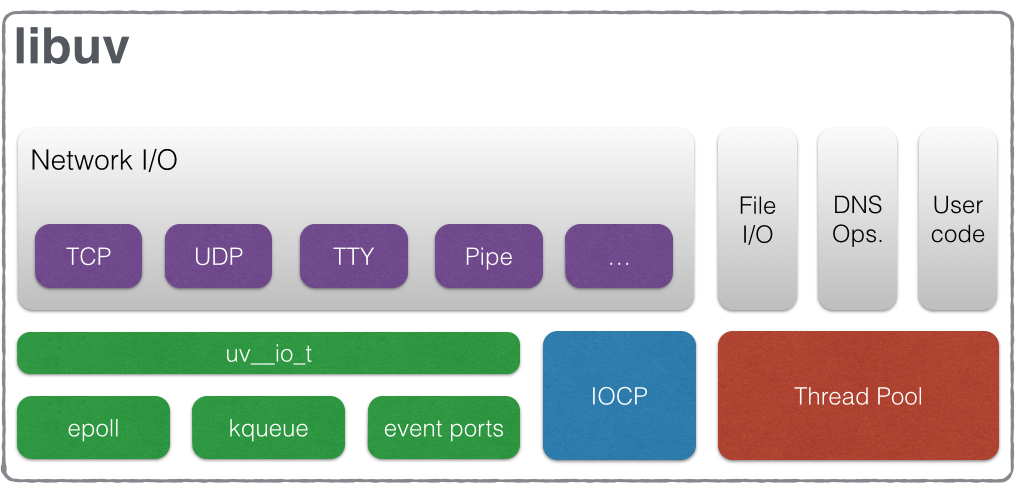
But what is a thread and how it’s different than a process? There are several differences, but the most important one for us is how the memory is allocated to them. You can think about a process like about an application. Inside each process, there is a chunk of memory dedicated just for this process. So, one process doesn`t have access to the memory of the second one, and this property ensures high security. To establish communication between them, we must do some work. Threads are different. Threads runs inside a process and share the same memory so there is no problem at all with threads sharing data.
However, one issue causes a problem. It’s called the race condition. The threads can run at the same time, so how do we know which ends first? It may happen that the first time you run it, the first operation ends first, and next time it may turn out to be the opposite and the second operation ends before the first one. Imagine working with write/read operations under such conditions! A nightmare! It’s sometimes very hard to write correct code in a multi-threaded environment.
Also, the multi-threaded languages have a large memory overhead because they create a separate thread for each request; so, if you want to call 1000 requests, they create 1000 threads.
How to deal with such a problem? Use a single thread instead! And that is what Node offers you.
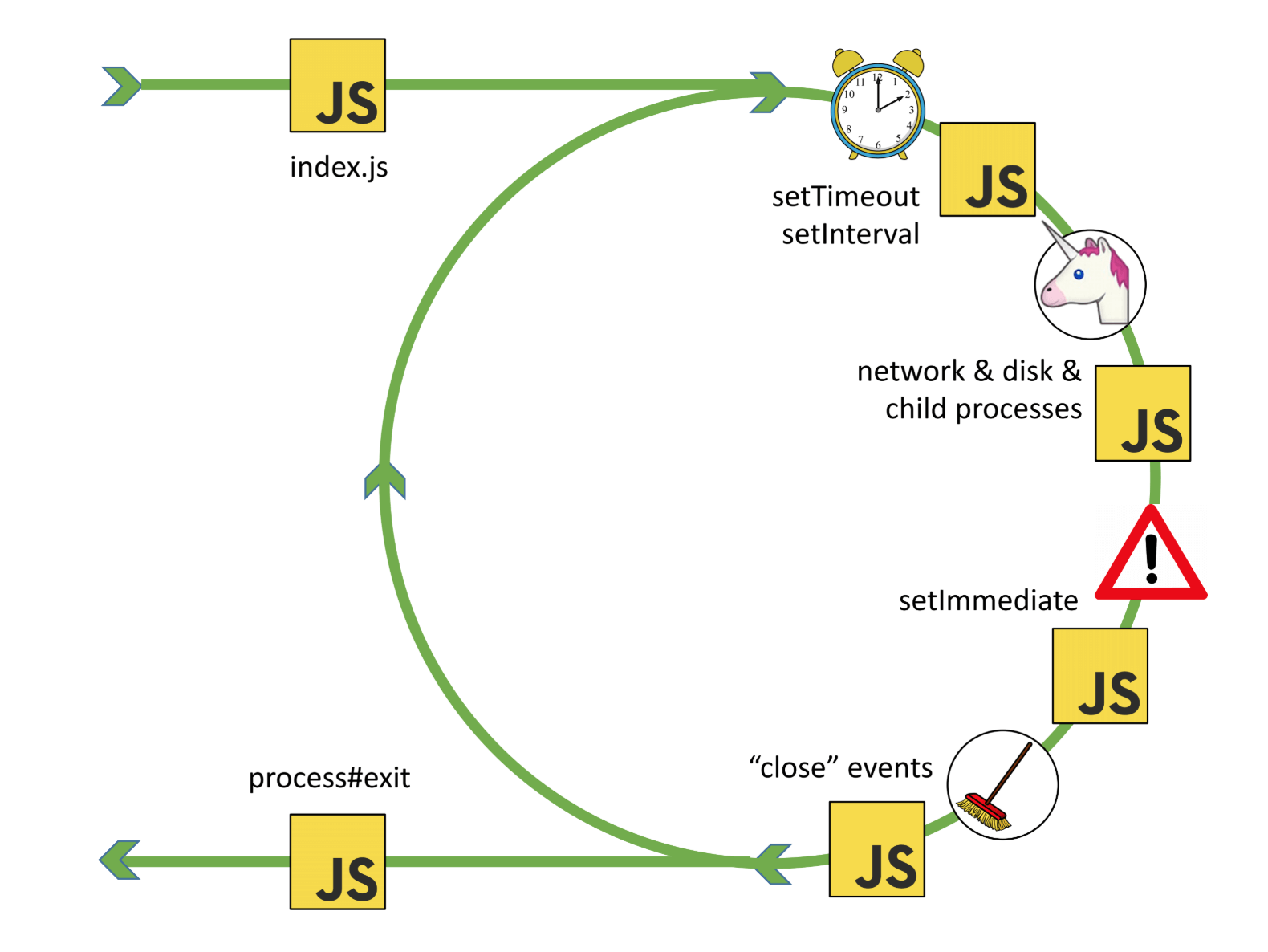
As a JavaScript developer I encourage you to watch the movie
in which Bart Belder clearly explains the concept of the event loop. The above diagram is taken from his presentation. And if you don’t know these terms at all, both Node and Libuv have excellent documentation 🙂
About blocking
In JavaScript development industry they say that because Node is single-threaded and non-blocking, you can achieve higher concurrency with the same resources than with multi-threaded solutions. It`s true but it is not as beautiful and easy as it may seem.
Since Node.js is single-threaded (JS part), CPU-intensive tasks will block all requests in progress until the particular task is completed. So, it`s true that in Node.js you can block every request just because one of them had a blocking instruction inside. The blocking code means that it takes more that few milliseconds to finish. But do not confuse long response time with blocking. The response from database can take a very long time, but it does not block your process (application).
Blocking methods execute synchronously and non-blocking methods execute asynchronously.
How can you slow down (or block) your event loop?
- vulnerable regex – a vulnerable regular expression is the one on which your regular expression engine might take exponential time; you can read mare about them here,
- JSON operations on large objects,
- using synchronous APIs from Node core modules instead of async versions; all of the I/O methods in the Node.js standard library provide also their asynchronous versions,
- other programming errors, like synchronous infinite loops.
In that case, since the Worker Pool uses a pool of threads, is it possible to block them too? Unfortunately, yes 🙁 Node is based on a philosophy one thread for many clients.
Let’s assume that a given task performed by a specific Worker is very complex and needs more time to be finished. As a result, the Worker is blocked and it can’t be used to execute any of the other pending tasks until its instructions are executed. As you have probably guessed by now, it may affect the performance. You can prevent such issues by minimizing the variation in Task times by using Task partitioning.
Conclusion
Avoid blocking, that’s for sure. If you only can, always choose asynchronous versions of the standard library APIs. Otherwise, after running your app, the client can experience several problems, starting with the degraded throughput and ending on complete withdrawal, which is fatal from the user’s perspective.
Read more:
Why you should (probably) use Typescript
How not to kill a project with bad coding practices?
Data fetching strategies in NextJS