PHP Development. Symfony Console Component – Tips & Tricks
This article was created with the aim to show you the most useful and retrieving tips and tricks about Symfony Console Development.
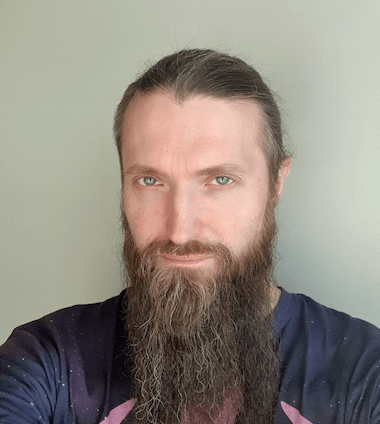
More than one article has been written about the mistakes made during the process of running a project, but rarely does one look at the project requirements and manage the risks given the technology chosen.
PHP, like other languages, has some drawbacks that are worth nothing when it comes to managing an IT project where PHP is the leading language.
Below we’ve curated a list of those, along with tips how to avoid them.
PHP is considered an “easy language” because it has a very low barrier to entry. This results is a large disparity in coding standards and how global interfaces are implemented in various external libraries. In an effort to bring order, a set of standards was introduced. These describe a set of ways in which the developer of implementation can satisfy any set of constraints required by the standard. A simple example for Event Dispatcher:
Listener – A Listener is any PHP callable that expects to be passed an Event. Zero or more Listeners may be passed the same Event. A Listener MAY enqueue some other asynchronous behavior if it so chooses.
Using this standard every developer using PSR-compiant nomenclature can easily not only communicate with but also code with other developers.
Putting these standards into practice, for example by using PHP The Right Way guidelines and libraries that support global PSR interfaces, allows PHP developers to adapt more quickly to changes in functional, architectural, and infrastructure requirements.
As a maintainer of the codebase always remember to use proven and stable versions of external libraries and if you’re forced to use custom-based solution implement it using PHP PSR’s.
A list of all available standards is available on the main website PHP-FIG. Extended standards with practical descriptions are available in a variety of formats from the PHP The Right Way homepage.
The best libraries that conform to the PHP-FIG standards are listed on the PHP League website.
In projects that use dependency manager Composer there is often a situation when after a long period of support and maintenance of the product in the production environment, there is a need to implement functional changes without rebuilding the whole architecture. Most often the project is then taken over by a programmer, whose task is to launch the local development environment and start working on tickets. Based on the composer.lock
file, the developer is able to restore the project to the state in which it was in the production environment, but any change to the composer.json
file, e.g. by adding the library, will cause a cascade of errors which will increase the actual time of implementation of a new team member to the organization as well as the time of development of the solution.
Once the application is stable, the code maintainer should lock down the versions of the libraries in the composer.json
file and create a clear procedure describing how to upgrade their versions if needed in the future.
Also consider running a mechanism to check the security status of the libraries used and automate the process of providing security updates.
Using free tools such as Dependabot, we can both maintain a consistent, manageable versioning infrastructure for dependent libraries and provide a security guarantee for our application.
> It’s just a CRUD, why bother?
> There’a a library that does exactly that!
In the PHP domain, it is easy to fall into the vortex of forgetfulness when implementing product business logic. Over the years there have been hundreds of projects that [create administrative panels to manage data models](https://backpackforlaravel.com/), [generate Google Analytics-like views](https://github.com/Kunstmaan/KunstmaanDashboardBundle) or [solve PHP’s async problems](https://laravel.com/docs/9.x/octane) at the touch of a magic wand (OK, with a single command line query).
The PHP world is full of ready-made implementations that work 99.9% of the time.
That 0.1% is where business logic collides with the functional limitations of the libraries used.
It’s these so-called ” throw-ins” that are the most difficult to implement at the end of the project.
There is no chance to find the golden mean between overengineering and the under engineering without proper understanding of the product’s business domain.
By starting development team members early in the product phase and being proactive while working with the product owner, you can minimize the risk of the problem of using a solution that won’t work as a long-term investment.
PHP is not perfect, that’s for sure. Its shortcomings in terms of static typing, lack of generics support, and continued support for archaic methods are still a source of jokes among programmers. However, for some time PHP developers have been given more and more powerful tools like PHPStan, Xdebug, PHP-CS-Fixer that allow them to maintain consistency and static typing – thus avoiding many bugs. Still, too little attention is paid to tests and those, when implemented correctly, result in a quick return on investment in the form of
– reduction of regression errors
– increased awareness of product capabilities
– increasing developer’s sense of code ownership
Don’t cut costs on testing. Writing simple Behat scripts really isn’t that hard, you don’t have to write complex end-to-end tests right away or get into implementation details and unit test every method.
A simple Behat test described in natural domain language is often worth more than the most meticulously written end-to-end test.
The PHP language and its two most powerful frameworks Laravel and Symfony are fully suitable for creating a modern, functional, and most importantly high performance architecture. The support for various message queue systems and the ever-faster PHP performance improvements from version to version allows us to easily create microservices based on microframeworks. For the most part, however, we still rely on monolithic systems. There’s nothing wrong with that, but when considering the development of such systems we need to pay close attention to the domain boundaries and determine the point of interface between new solutions and older parts of the system.
When developing any PHP website, it is worthwhile to take a close look at current solutions, create global interfaces for data communication and implement new functionalities using the latest techniques and practices. One of the most popular solutions used in practice is Strangler Pattern.